Integrating external data from a CSV 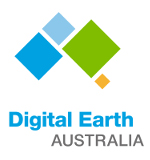
Sign up to the DEA Sandbox to run this notebook interactively from a browser
Compatibility: Notebook currently compatible with both the
NCI
andDEA Sandbox
environmentsProducts used: ga_ls8c_ard_3
Background
It is often useful to combine external data (e.g. from a CSV or other dataset) with data loaded from Digital Earth Australia. For example, we may want to combine data from a tide or river gauge with satellite data to determine water levels at the exact moment each satellite observation was made. This can allow us to manipulate and extract satellite data and obtain additional insights using data from our external source.
Description
This example notebook loads in a time series of external tide modelling data from a CSV, and combines it with satellite data loaded from Digital Earth Australia. This workflow could be applied to any external time series dataset (e.g. river gauges, tide guagues, rainfall measurements etc). The notebook demonstrates how to:
Load a time series of Landsat 8 data
Load in external time series data from a CSV
Convert this data to an
xarray.Dataset
and link it to each satellite observation by interpolating values at each satellite timestepAdd this new data as a variable in our satellite dataset, and use this to filter satellite imagery to high and low tide imagery
Demonstrate how to swap dimensions between
time
and the newtide_height
variable
Getting started
To run this analysis, run all the cells in the notebook, starting with the “Load packages” cell.
Load packages
[1]:
%matplotlib inline
import datacube
import pandas as pd
import matplotlib.pyplot as plt
pd.plotting.register_matplotlib_converters()
Connect to the datacube
[2]:
dc = datacube.Datacube(app='Integrating_external_data')
Loading satellite data
First we load in a year of Landsat 8 data. We will use Moreton Bay in Queensland for this demonstration, as we have a CSV of tide height data for this location that we wish to combine with satellite data. We load a single band nbart_nir
which clearly differentiates between water (low values) and land (higher values). This will let us verify that we can use external tide data to identify low and high tide satellite observations.
[3]:
# Set up a location for the analysis
query = {
'x': (153.38, 153.42),
'y': (-27.63, -27.67),
'time': ('2015-01-01', '2015-12-31'),
'measurements': ['nbart_nir'],
'output_crs': 'EPSG:3577',
'resolution': (-30, 30)
}
# Load Landsat 8 data
ds = dc.load(product='ga_ls8c_ard_3', group_by='solar_day', **query)
ds
[3]:
<xarray.Dataset> Dimensions: (time: 23, x: 154, y: 170) Coordinates: * time (time) datetime64[ns] 2015-01-11T23:42:10.081625 ... 2015-12... * y (y) float64 -3.171e+06 -3.172e+06 ... -3.177e+06 -3.177e+06 * x (x) float64 2.074e+06 2.074e+06 ... 2.078e+06 2.078e+06 spatial_ref int32 3577 Data variables: nbart_nir (time, y, x) int16 6511 6509 6451 6434 ... 1976 1967 2150 2254 Attributes: crs: EPSG:3577 grid_mapping: spatial_ref
- time: 23
- x: 154
- y: 170
- time(time)datetime64[ns]2015-01-11T23:42:10.081625 ... 2...
- units :
- seconds since 1970-01-01 00:00:00
array(['2015-01-11T23:42:10.081625000', '2015-01-27T23:42:04.551305000', '2015-02-12T23:41:56.406130000', '2015-02-28T23:41:53.631747000', '2015-03-16T23:41:42.785277000', '2015-04-01T23:41:30.942739000', '2015-04-17T23:41:30.125822000', '2015-05-03T23:41:17.918949000', '2015-05-19T23:41:09.909015000', '2015-06-04T23:41:17.891768000', '2015-06-20T23:41:26.191518000', '2015-07-06T23:41:35.434452000', '2015-07-22T23:41:42.800136000', '2015-08-07T23:41:45.488548000', '2015-08-23T23:41:53.807518000', '2015-09-08T23:41:59.816227000', '2015-09-24T23:42:06.978815000', '2015-10-10T23:42:08.144421000', '2015-10-26T23:42:12.986143000', '2015-11-11T23:42:13.292036000', '2015-11-27T23:42:16.500346000', '2015-12-13T23:42:14.793025000', '2015-12-29T23:42:14.365276000'], dtype='datetime64[ns]')
- y(y)float64-3.171e+06 ... -3.177e+06
- units :
- metre
- resolution :
- -30.0
- crs :
- EPSG:3577
array([-3171495., -3171525., -3171555., -3171585., -3171615., -3171645., -3171675., -3171705., -3171735., -3171765., -3171795., -3171825., -3171855., -3171885., -3171915., -3171945., -3171975., -3172005., -3172035., -3172065., -3172095., -3172125., -3172155., -3172185., -3172215., -3172245., -3172275., -3172305., -3172335., -3172365., -3172395., -3172425., -3172455., -3172485., -3172515., -3172545., -3172575., -3172605., -3172635., -3172665., -3172695., -3172725., -3172755., -3172785., -3172815., -3172845., -3172875., -3172905., -3172935., -3172965., -3172995., -3173025., -3173055., -3173085., -3173115., -3173145., -3173175., -3173205., -3173235., -3173265., -3173295., -3173325., -3173355., -3173385., -3173415., -3173445., -3173475., -3173505., -3173535., -3173565., -3173595., -3173625., -3173655., -3173685., -3173715., -3173745., -3173775., -3173805., -3173835., -3173865., -3173895., -3173925., -3173955., -3173985., -3174015., -3174045., -3174075., -3174105., -3174135., -3174165., -3174195., -3174225., -3174255., -3174285., -3174315., -3174345., -3174375., -3174405., -3174435., -3174465., -3174495., -3174525., -3174555., -3174585., -3174615., -3174645., -3174675., -3174705., -3174735., -3174765., -3174795., -3174825., -3174855., -3174885., -3174915., -3174945., -3174975., -3175005., -3175035., -3175065., -3175095., -3175125., -3175155., -3175185., -3175215., -3175245., -3175275., -3175305., -3175335., -3175365., -3175395., -3175425., -3175455., -3175485., -3175515., -3175545., -3175575., -3175605., -3175635., -3175665., -3175695., -3175725., -3175755., -3175785., -3175815., -3175845., -3175875., -3175905., -3175935., -3175965., -3175995., -3176025., -3176055., -3176085., -3176115., -3176145., -3176175., -3176205., -3176235., -3176265., -3176295., -3176325., -3176355., -3176385., -3176415., -3176445., -3176475., -3176505., -3176535., -3176565.])
- x(x)float642.074e+06 2.074e+06 ... 2.078e+06
- units :
- metre
- resolution :
- 30.0
- crs :
- EPSG:3577
array([2073825., 2073855., 2073885., 2073915., 2073945., 2073975., 2074005., 2074035., 2074065., 2074095., 2074125., 2074155., 2074185., 2074215., 2074245., 2074275., 2074305., 2074335., 2074365., 2074395., 2074425., 2074455., 2074485., 2074515., 2074545., 2074575., 2074605., 2074635., 2074665., 2074695., 2074725., 2074755., 2074785., 2074815., 2074845., 2074875., 2074905., 2074935., 2074965., 2074995., 2075025., 2075055., 2075085., 2075115., 2075145., 2075175., 2075205., 2075235., 2075265., 2075295., 2075325., 2075355., 2075385., 2075415., 2075445., 2075475., 2075505., 2075535., 2075565., 2075595., 2075625., 2075655., 2075685., 2075715., 2075745., 2075775., 2075805., 2075835., 2075865., 2075895., 2075925., 2075955., 2075985., 2076015., 2076045., 2076075., 2076105., 2076135., 2076165., 2076195., 2076225., 2076255., 2076285., 2076315., 2076345., 2076375., 2076405., 2076435., 2076465., 2076495., 2076525., 2076555., 2076585., 2076615., 2076645., 2076675., 2076705., 2076735., 2076765., 2076795., 2076825., 2076855., 2076885., 2076915., 2076945., 2076975., 2077005., 2077035., 2077065., 2077095., 2077125., 2077155., 2077185., 2077215., 2077245., 2077275., 2077305., 2077335., 2077365., 2077395., 2077425., 2077455., 2077485., 2077515., 2077545., 2077575., 2077605., 2077635., 2077665., 2077695., 2077725., 2077755., 2077785., 2077815., 2077845., 2077875., 2077905., 2077935., 2077965., 2077995., 2078025., 2078055., 2078085., 2078115., 2078145., 2078175., 2078205., 2078235., 2078265., 2078295., 2078325., 2078355., 2078385., 2078415.])
- spatial_ref()int323577
- spatial_ref :
- PROJCS["GDA94 / Australian Albers",GEOGCS["GDA94",DATUM["Geocentric_Datum_of_Australia_1994",SPHEROID["GRS 1980",6378137,298.257222101,AUTHORITY["EPSG","7019"]],AUTHORITY["EPSG","6283"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4283"]],PROJECTION["Albers_Conic_Equal_Area"],PARAMETER["latitude_of_center",0],PARAMETER["longitude_of_center",132],PARAMETER["standard_parallel_1",-18],PARAMETER["standard_parallel_2",-36],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","3577"]]
- grid_mapping_name :
- albers_conical_equal_area
array(3577, dtype=int32)
- nbart_nir(time, y, x)int166511 6509 6451 ... 1967 2150 2254
- units :
- 1
- nodata :
- -999
- crs :
- EPSG:3577
- grid_mapping :
- spatial_ref
array([[[6511, 6509, 6451, ..., 7167, 6882, 6651], [6427, 6433, 6426, ..., 7298, 6951, 6748], [6326, 6362, 6421, ..., 7394, 7075, 6790], ..., [5496, 5500, 5526, ..., 6252, 6388, 6492], [5473, 5537, 5584, ..., 6212, 6302, 6428], [5390, 5491, 5553, ..., 6183, 6284, 6385]], [[7082, 7084, 7004, ..., 6339, 6186, 5999], [6968, 6945, 6947, ..., 6429, 6253, 6086], [6893, 6922, 6979, ..., 6555, 6326, 6146], ..., [8034, 8019, 7952, ..., 5707, 5781, 5969], [7972, 7954, 7914, ..., 5604, 5668, 5862], [7879, 7874, 7827, ..., 5544, 5606, 5731]], [[6161, 5418, 4691, ..., 2286, 2469, 2781], [6202, 6121, 5411, ..., 2453, 2492, 2590], [6441, 5756, 4958, ..., 2434, 2595, 2680], ..., ... ..., [6558, 6322, 6203, ..., 2669, 2568, 2612], [6337, 6486, 6313, ..., 2585, 2527, 2676], [5976, 6298, 6344, ..., 2735, 2526, 2684]], [[1301, 2262, 2480, ..., 6600, 6792, 6888], [1747, 2663, 2887, ..., 6442, 6734, 6915], [2884, 3044, 3611, ..., 6320, 6641, 6568], ..., [4668, 4286, 4268, ..., 1839, 1912, 1914], [4630, 4764, 4910, ..., 1785, 1991, 1987], [4684, 4842, 5272, ..., 1778, 1896, 1967]], [[ 437, 363, 649, ..., 1916, 1858, 2340], [ 282, 282, 288, ..., 2022, 2069, 2271], [ 296, 294, 324, ..., 1976, 2192, 2432], ..., [2427, 2497, 2441, ..., 2055, 2161, 2095], [2314, 2441, 2411, ..., 1978, 2228, 2234], [2356, 2417, 2311, ..., 1967, 2150, 2254]]], dtype=int16)
- crs :
- EPSG:3577
- grid_mapping :
- spatial_ref
Integrating external data
Load in a CSV of external data with timestamps
In the code below, we aim to take a CSV file of external data (in this case, half-hourly tide heights for a location in Moreton Bay, Queensland for a five year period between 2014 and 2018 generated using the OTPS TPXO8 tidal model), and link this data back to our satellite data timeseries.
We can load the existing tide height data using the pandas
module which we imported earlier. The data has a column of time
values, which we will set as the index column (roughly equivelent to a dimension
in xarray
).
[4]:
tide_data = pd.read_csv('../Supplementary_data/Integrating_external_data/moretonbay_-27.55_153.35_2014-2018_tides.csv',
parse_dates=['time'],
index_col='time')
tide_data.head()
[4]:
tide_height | |
---|---|
time | |
2014-01-01 00:00:00 | 1.179 |
2014-01-01 00:30:00 | 1.012 |
2014-01-01 01:00:00 | 0.805 |
2014-01-01 01:30:00 | 0.569 |
2014-01-01 02:00:00 | 0.316 |
Link external data to satellite data
Now that we have the tide height data, we need to estimate the tide height for each of our satellite images. We can do this by interpolating between the data points we do have (hourly measurements) to get an estimated tide height for the exact moment each satellite image was taken.
The code below generates an xarray.Dataset
with the same number of timesteps as the original satellite data. The dataset has a single variable tide_height
that gives the estimated tide hight for each observation:
[5]:
# First, we convert the data to an xarray dataset so we can analyse it
# in the same way as oursatellite data
tide_data_xr = tide_data.to_xarray()
# We want to convert our hourly tide heights to estimates of exactly how
# high the tide was at the time that each satellite image was taken. To
# do this, we can use `.interp` to 'interpolate' a tide height for each
# satellite timestamp:
satellite_tideheights = tide_data_xr.interp(time=ds.time)
# Print the output xarray object
satellite_tideheights
[5]:
<xarray.Dataset> Dimensions: (time: 23) Coordinates: * time (time) datetime64[ns] 2015-01-11T23:42:10.081625 ... 2015-12... spatial_ref int32 3577 Data variables: tide_height (time) float64 0.02013 -0.2608 -0.2658 ... 1.105 1.007 0.4098
- time: 23
- time(time)datetime64[ns]2015-01-11T23:42:10.081625 ... 2...
- units :
- seconds since 1970-01-01 00:00:00
array(['2015-01-11T23:42:10.081625000', '2015-01-27T23:42:04.551305000', '2015-02-12T23:41:56.406130000', '2015-02-28T23:41:53.631747000', '2015-03-16T23:41:42.785277000', '2015-04-01T23:41:30.942739000', '2015-04-17T23:41:30.125822000', '2015-05-03T23:41:17.918949000', '2015-05-19T23:41:09.909015000', '2015-06-04T23:41:17.891768000', '2015-06-20T23:41:26.191518000', '2015-07-06T23:41:35.434452000', '2015-07-22T23:41:42.800136000', '2015-08-07T23:41:45.488548000', '2015-08-23T23:41:53.807518000', '2015-09-08T23:41:59.816227000', '2015-09-24T23:42:06.978815000', '2015-10-10T23:42:08.144421000', '2015-10-26T23:42:12.986143000', '2015-11-11T23:42:13.292036000', '2015-11-27T23:42:16.500346000', '2015-12-13T23:42:14.793025000', '2015-12-29T23:42:14.365276000'], dtype='datetime64[ns]')
- spatial_ref()int323577
- spatial_ref :
- PROJCS["GDA94 / Australian Albers",GEOGCS["GDA94",DATUM["Geocentric_Datum_of_Australia_1994",SPHEROID["GRS 1980",6378137,298.257222101,AUTHORITY["EPSG","7019"]],AUTHORITY["EPSG","6283"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4283"]],PROJECTION["Albers_Conic_Equal_Area"],PARAMETER["latitude_of_center",0],PARAMETER["longitude_of_center",132],PARAMETER["standard_parallel_1",-18],PARAMETER["standard_parallel_2",-36],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","3577"]]
- grid_mapping_name :
- albers_conical_equal_area
array(3577, dtype=int32)
- tide_height(time)float640.02013 -0.2608 ... 1.007 0.4098
array([ 0.02013372, -0.2608177 , -0.26579601, 0.16721747, 0.16303624, 0.4644923 , 0.77548951, 0.56624689, 0.46198076, 0.38001156, -0.04782294, -0.31538832, -0.33561288, -0.63533705, -0.53267225, -0.2105904 , -0.25798545, 0.35483894, 0.91898997, 0.91977909, 1.10450752, 1.00684373, 0.40984451])
Now we have our tide heights in xarray
format that matches our satellite data, we can add this back into our satellite dataset as a new variable:
[6]:
# We then want to put these values back into the Landsat dataset so
# that each image has an estimated tide height:
ds['tide_height'] = satellite_tideheights.tide_height
We can now plot tide heights for our satellite data:
[7]:
# Plot the resulting tide heights for each Landsat image:
ds.tide_height.plot()
plt.show()
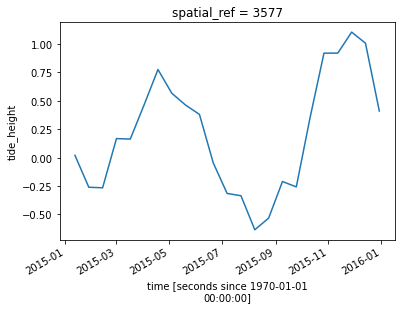
Filtering by external data
Now that we have a new variable tide_height
in our dataset, we can use xarray
indexing methods to manipulate our data using tide heights (e.g. filter by tide to select low or high tide images):
[8]:
# Select a subset of low tide images (tide less than -0.6 m)
low_tide_ds = ds.sel(time = ds.tide_height < -0.60)
# Select a subset of high tide images (tide greater than 0.9 m)
high_tide_ds = ds.sel(time = ds.tide_height > 0.9)
We can plot an image from these subsets to verify that the satellite images were observed at low tide:
[9]:
low_tide_ds.isel(time=0).nbart_nir.plot(robust=True)
plt.title("Low tide")
plt.show()
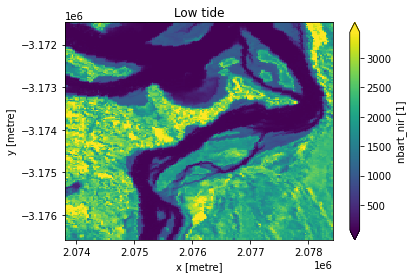
And at high tide. Note that many tidal flat areas in the image above (blue-green) now appear inundated by water (dark blue/purple):
[10]:
high_tide_ds.isel(time=0).nbart_nir.plot(robust=True)
plt.title("High tide")
plt.show()
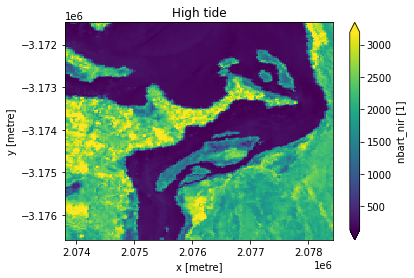
Swapping dimensions based on external data
By default, xarray
uses time
as one of the main dimensions in the dataset (in addition to x
and y
). Now that we have a new tide_height
variable, we can change this to be an actual dimension in the dataset in place of time
. This enables additional more advanced operations, such as calculating rolling statistics or aggregating by tide_heights
.
In the example below, you can see that the dataset now has three dimensions (tide_height
, x
and y
). The dimension time
is no longer a dimension in the dataset.
[11]:
ds.swap_dims({'time': 'tide_height'})
[11]:
<xarray.Dataset> Dimensions: (tide_height: 23, x: 154, y: 170) Coordinates: time (tide_height) datetime64[ns] 2015-01-11T23:42:10.081625 ... ... * y (y) float64 -3.171e+06 -3.172e+06 ... -3.177e+06 -3.177e+06 * x (x) float64 2.074e+06 2.074e+06 ... 2.078e+06 2.078e+06 spatial_ref int32 3577 * tide_height (tide_height) float64 0.02013 -0.2608 -0.2658 ... 1.007 0.4098 Data variables: nbart_nir (tide_height, y, x) int16 6511 6509 6451 ... 1967 2150 2254 Attributes: crs: EPSG:3577 grid_mapping: spatial_ref
- tide_height: 23
- x: 154
- y: 170
- time(tide_height)datetime64[ns]2015-01-11T23:42:10.081625 ... 2...
- units :
- seconds since 1970-01-01 00:00:00
array(['2015-01-11T23:42:10.081625000', '2015-01-27T23:42:04.551305000', '2015-02-12T23:41:56.406130000', '2015-02-28T23:41:53.631747000', '2015-03-16T23:41:42.785277000', '2015-04-01T23:41:30.942739000', '2015-04-17T23:41:30.125822000', '2015-05-03T23:41:17.918949000', '2015-05-19T23:41:09.909015000', '2015-06-04T23:41:17.891768000', '2015-06-20T23:41:26.191518000', '2015-07-06T23:41:35.434452000', '2015-07-22T23:41:42.800136000', '2015-08-07T23:41:45.488548000', '2015-08-23T23:41:53.807518000', '2015-09-08T23:41:59.816227000', '2015-09-24T23:42:06.978815000', '2015-10-10T23:42:08.144421000', '2015-10-26T23:42:12.986143000', '2015-11-11T23:42:13.292036000', '2015-11-27T23:42:16.500346000', '2015-12-13T23:42:14.793025000', '2015-12-29T23:42:14.365276000'], dtype='datetime64[ns]')
- y(y)float64-3.171e+06 ... -3.177e+06
- units :
- metre
- resolution :
- -30.0
- crs :
- EPSG:3577
array([-3171495., -3171525., -3171555., -3171585., -3171615., -3171645., -3171675., -3171705., -3171735., -3171765., -3171795., -3171825., -3171855., -3171885., -3171915., -3171945., -3171975., -3172005., -3172035., -3172065., -3172095., -3172125., -3172155., -3172185., -3172215., -3172245., -3172275., -3172305., -3172335., -3172365., -3172395., -3172425., -3172455., -3172485., -3172515., -3172545., -3172575., -3172605., -3172635., -3172665., -3172695., -3172725., -3172755., -3172785., -3172815., -3172845., -3172875., -3172905., -3172935., -3172965., -3172995., -3173025., -3173055., -3173085., -3173115., -3173145., -3173175., -3173205., -3173235., -3173265., -3173295., -3173325., -3173355., -3173385., -3173415., -3173445., -3173475., -3173505., -3173535., -3173565., -3173595., -3173625., -3173655., -3173685., -3173715., -3173745., -3173775., -3173805., -3173835., -3173865., -3173895., -3173925., -3173955., -3173985., -3174015., -3174045., -3174075., -3174105., -3174135., -3174165., -3174195., -3174225., -3174255., -3174285., -3174315., -3174345., -3174375., -3174405., -3174435., -3174465., -3174495., -3174525., -3174555., -3174585., -3174615., -3174645., -3174675., -3174705., -3174735., -3174765., -3174795., -3174825., -3174855., -3174885., -3174915., -3174945., -3174975., -3175005., -3175035., -3175065., -3175095., -3175125., -3175155., -3175185., -3175215., -3175245., -3175275., -3175305., -3175335., -3175365., -3175395., -3175425., -3175455., -3175485., -3175515., -3175545., -3175575., -3175605., -3175635., -3175665., -3175695., -3175725., -3175755., -3175785., -3175815., -3175845., -3175875., -3175905., -3175935., -3175965., -3175995., -3176025., -3176055., -3176085., -3176115., -3176145., -3176175., -3176205., -3176235., -3176265., -3176295., -3176325., -3176355., -3176385., -3176415., -3176445., -3176475., -3176505., -3176535., -3176565.])
- x(x)float642.074e+06 2.074e+06 ... 2.078e+06
- units :
- metre
- resolution :
- 30.0
- crs :
- EPSG:3577
array([2073825., 2073855., 2073885., 2073915., 2073945., 2073975., 2074005., 2074035., 2074065., 2074095., 2074125., 2074155., 2074185., 2074215., 2074245., 2074275., 2074305., 2074335., 2074365., 2074395., 2074425., 2074455., 2074485., 2074515., 2074545., 2074575., 2074605., 2074635., 2074665., 2074695., 2074725., 2074755., 2074785., 2074815., 2074845., 2074875., 2074905., 2074935., 2074965., 2074995., 2075025., 2075055., 2075085., 2075115., 2075145., 2075175., 2075205., 2075235., 2075265., 2075295., 2075325., 2075355., 2075385., 2075415., 2075445., 2075475., 2075505., 2075535., 2075565., 2075595., 2075625., 2075655., 2075685., 2075715., 2075745., 2075775., 2075805., 2075835., 2075865., 2075895., 2075925., 2075955., 2075985., 2076015., 2076045., 2076075., 2076105., 2076135., 2076165., 2076195., 2076225., 2076255., 2076285., 2076315., 2076345., 2076375., 2076405., 2076435., 2076465., 2076495., 2076525., 2076555., 2076585., 2076615., 2076645., 2076675., 2076705., 2076735., 2076765., 2076795., 2076825., 2076855., 2076885., 2076915., 2076945., 2076975., 2077005., 2077035., 2077065., 2077095., 2077125., 2077155., 2077185., 2077215., 2077245., 2077275., 2077305., 2077335., 2077365., 2077395., 2077425., 2077455., 2077485., 2077515., 2077545., 2077575., 2077605., 2077635., 2077665., 2077695., 2077725., 2077755., 2077785., 2077815., 2077845., 2077875., 2077905., 2077935., 2077965., 2077995., 2078025., 2078055., 2078085., 2078115., 2078145., 2078175., 2078205., 2078235., 2078265., 2078295., 2078325., 2078355., 2078385., 2078415.])
- spatial_ref()int323577
- spatial_ref :
- PROJCS["GDA94 / Australian Albers",GEOGCS["GDA94",DATUM["Geocentric_Datum_of_Australia_1994",SPHEROID["GRS 1980",6378137,298.257222101,AUTHORITY["EPSG","7019"]],AUTHORITY["EPSG","6283"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4283"]],PROJECTION["Albers_Conic_Equal_Area"],PARAMETER["latitude_of_center",0],PARAMETER["longitude_of_center",132],PARAMETER["standard_parallel_1",-18],PARAMETER["standard_parallel_2",-36],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","3577"]]
- grid_mapping_name :
- albers_conical_equal_area
array(3577, dtype=int32)
- tide_height(tide_height)float640.02013 -0.2608 ... 1.007 0.4098
array([ 0.020134, -0.260818, -0.265796, 0.167217, 0.163036, 0.464492, 0.77549 , 0.566247, 0.461981, 0.380012, -0.047823, -0.315388, -0.335613, -0.635337, -0.532672, -0.21059 , -0.257985, 0.354839, 0.91899 , 0.919779, 1.104508, 1.006844, 0.409845])
- nbart_nir(tide_height, y, x)int166511 6509 6451 ... 1967 2150 2254
- units :
- 1
- nodata :
- -999
- crs :
- EPSG:3577
- grid_mapping :
- spatial_ref
array([[[6511, 6509, 6451, ..., 7167, 6882, 6651], [6427, 6433, 6426, ..., 7298, 6951, 6748], [6326, 6362, 6421, ..., 7394, 7075, 6790], ..., [5496, 5500, 5526, ..., 6252, 6388, 6492], [5473, 5537, 5584, ..., 6212, 6302, 6428], [5390, 5491, 5553, ..., 6183, 6284, 6385]], [[7082, 7084, 7004, ..., 6339, 6186, 5999], [6968, 6945, 6947, ..., 6429, 6253, 6086], [6893, 6922, 6979, ..., 6555, 6326, 6146], ..., [8034, 8019, 7952, ..., 5707, 5781, 5969], [7972, 7954, 7914, ..., 5604, 5668, 5862], [7879, 7874, 7827, ..., 5544, 5606, 5731]], [[6161, 5418, 4691, ..., 2286, 2469, 2781], [6202, 6121, 5411, ..., 2453, 2492, 2590], [6441, 5756, 4958, ..., 2434, 2595, 2680], ..., ... ..., [6558, 6322, 6203, ..., 2669, 2568, 2612], [6337, 6486, 6313, ..., 2585, 2527, 2676], [5976, 6298, 6344, ..., 2735, 2526, 2684]], [[1301, 2262, 2480, ..., 6600, 6792, 6888], [1747, 2663, 2887, ..., 6442, 6734, 6915], [2884, 3044, 3611, ..., 6320, 6641, 6568], ..., [4668, 4286, 4268, ..., 1839, 1912, 1914], [4630, 4764, 4910, ..., 1785, 1991, 1987], [4684, 4842, 5272, ..., 1778, 1896, 1967]], [[ 437, 363, 649, ..., 1916, 1858, 2340], [ 282, 282, 288, ..., 2022, 2069, 2271], [ 296, 294, 324, ..., 1976, 2192, 2432], ..., [2427, 2497, 2441, ..., 2055, 2161, 2095], [2314, 2441, 2411, ..., 1978, 2228, 2234], [2356, 2417, 2311, ..., 1967, 2150, 2254]]], dtype=int16)
- crs :
- EPSG:3577
- grid_mapping :
- spatial_ref
Additional information
License: The code in this notebook is licensed under the Apache License, Version 2.0. Digital Earth Australia data is licensed under the Creative Commons by Attribution 4.0 license.
Contact: If you need assistance, please post a question on the Open Data Cube Slack channel or on the GIS Stack Exchange using the open-data-cube
tag (you can view previously asked questions here). If you would like to report an issue with this notebook, you can file one on
GitHub.
Last modified: December 2023
Compatible datacube version:
[12]:
print(datacube.__version__)
1.8.5
Tags
Tags: sandbox compatible, NCI compatible, time series analysis, landsat 8, external data, interpolation, indexing, swapping dimensions, csv, intertidal, tide modelling